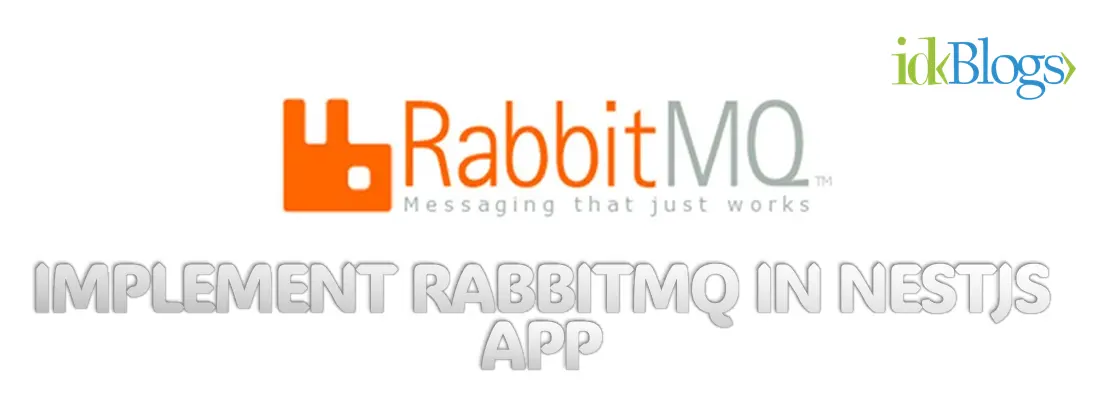
In this article, we'll create a CLI based application nodejs. It means you will interact only with the Node CLI.
in this we'll Create a CLI application to add two numbers in nodejs
Now we are going to make a simple add application to add number(s). We'll also implement the error handling logic
within this application.
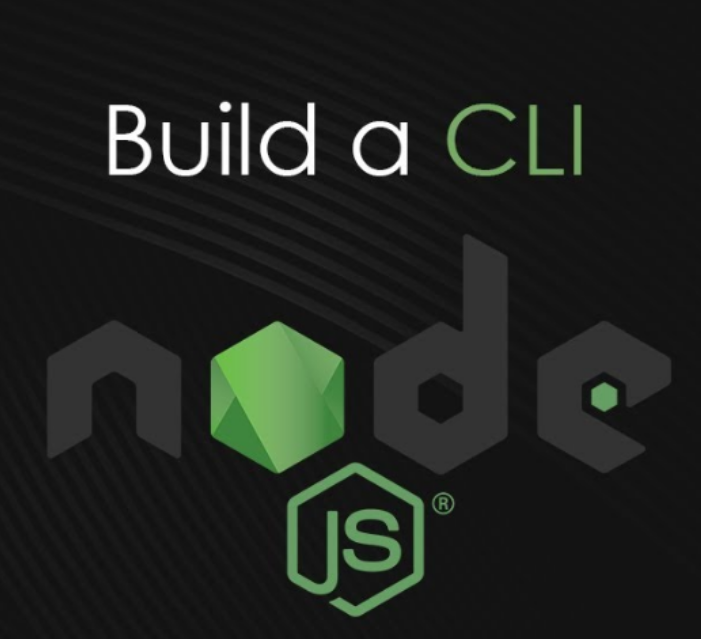
Create CLI application in nodejs | idkblogs.com
So let’s dive into the codes and create CLI application in NodeJS:
Create a file name "cliNodeApp.js" and start writing the below codes:Instantiate the readline module:
var readline = require(‘readline’);
The above module will help us to create an interface for CLI application.
Instantiate the CLI Module object:
var cli = {};
In the above code, we are creating a simple object "cli" without any property.
Now create the CLI functions as:
cli.processInput = function (str) {
str = typeof (str) == ‘string’ && str.trim().length > 0 ? str.trim() : false;
if (str) {
var inputs = str.split(‘ ‘);
if (inputs.length >= 3) {
var results = 0;
inputs.some(function (value) {
if (!isNaN(value)) {
results = results + parseInt(value);
}
})
console.log(“\x1b[36m”, ”Total is : ”, results);
return true;
} else {
console.error(“\x1b[31m”, ”Error: Too few parameter”);
return false;
}
}
}
Create init script to start the CLI application:
cli.init = function () {
/* Send the start message to the console in dark blue. */
console.log(‘\x1b[33m % s\x1b[0m’, ‘CLI is running’);
/* Start the interface */
var _interface = readline.createInterface({
input: process.stdin,
output: process.stdout,
prompt: ‘>’
})
/* Create an initial prompt */
_interface.prompt(); // Will wait for input /* Handle each line of input separately. */
_interface.on(‘line’, function (str) { /* Send to input processor. */
cli.processInput(str); /* re-initialize the prompt afterwards. */
_interface.prompt();
});
}
Now start the script:
cli.init();
Save the app:
Save this application as cliNodeApp.js
Run the application:
node cliNodeApp.js
Congratulations… you have created your CLI application in nodeJs.
Now you can take the idea from this codes and do whatever you want. This would be your basic nodejs CLI application.
Output:
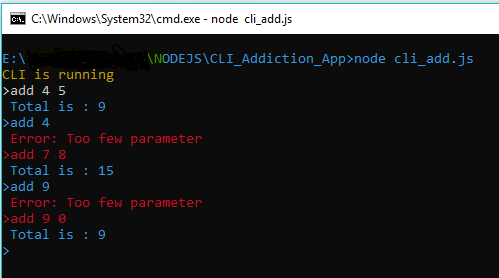
Create CLI application in nodejs | idkblogs.com
Here is the complete code ( Snapshot ):
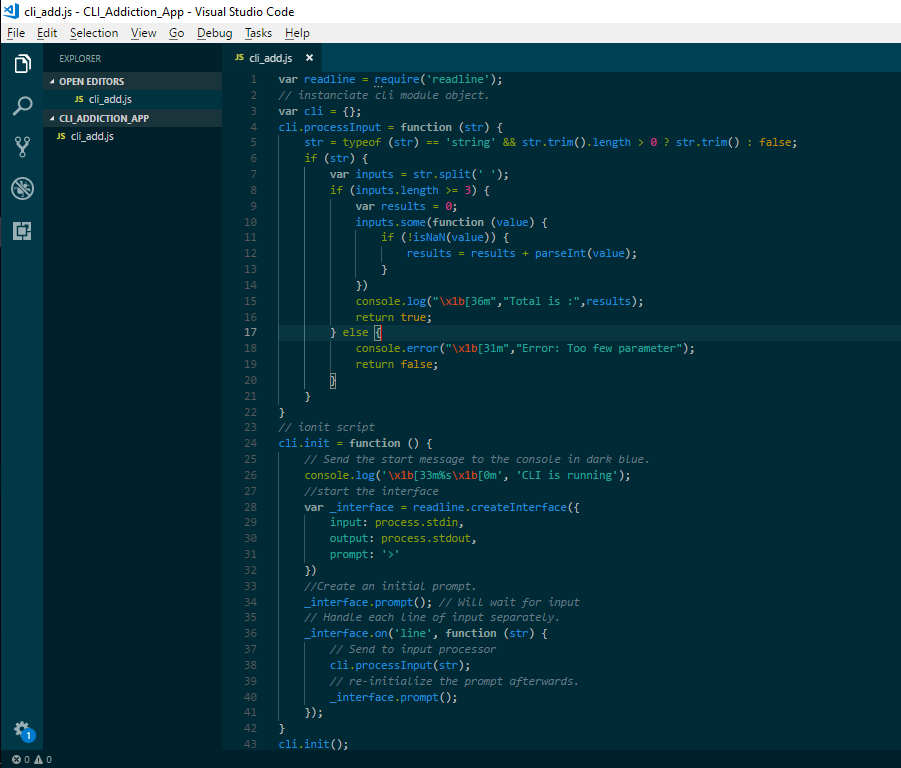
Create CLI application in nodejs | idkblogs.com
Conclusion:
In this article, we learned about the CLI app and how we can crate a node CLI app.
Support our IDKBlogs team
Creating quality content takes time and resources, and we are committed to providing value to our
readers.
If you find my articles helpful or informative, please consider supporting us financially.
Any amount (10, 20, 50, 100, ....), no matter how small, will help us continue to produce
high-quality content.
Thank you for your support!
Thank you
I appreciate you taking the time to read this article. The more that you read, the more things you will know. The more that you learn, the more places you'll go.
If you’re interested in Node.js or JavaScript this link will help you a lot.
If you found this article is helpful, then please share this article's link to your friends to whom this is required, you can share this to your technical social media groups also.
You can follow us on our social media page for more updates and latest article updates.
To read more about the technologies, Please
subscribe us, You'll get the monthly newsletter having all the published
article of the last month.