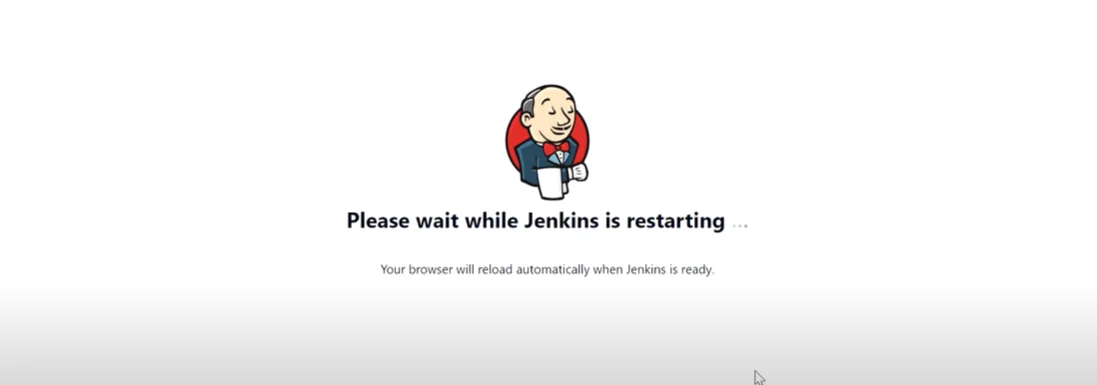
How to implement instance factory for multiple classes
An instance factory is a design pattern that encapsulates the creation of objects and allows for flexibility in instantiation. Implementing an instance factory can be quite useful when dealing with multiple classes, as it centralizes object creation and can simplify code maintenance.
Here's how you can implement an instance factory for multiple classes in TypeScript:
Define Interface:
If your all class have the same methods, then its a good idea to define the interface.ITestPremiumCalculators.ts
Define Classes:
Start by defining the classes for which you want to create instances using the factory.PlanA.ts
PlanB.ts
Create Factory:
Create a factory class that contains methods for creating instances of the different classesPlanFactory.ts
Usage:
Now you can use the instance factory to create instances of the classes in a centralized manner.
By using an instance factory, you can easily manage and maintain object creation for multiple classes. If you need
to modify the instantiation process or add common logic, you can do so within the factory without affecting the
client code that uses the factory.
Additionally, if you have a large number of classes or want more dynamic instantiation, you can use a more advanced approach like a registry or mapping to associate class names or identifiers with their corresponding creation methods in the factory.
implementing an instance factory pattern provides a structured and centralized way to create instances of multiple classes. This design pattern offers several advantages that contribute to cleaner, more maintainable, and flexible code:
-
Code Organization: The instance factory segregates object creation logic from the rest of the application, promoting better code organization and separation of concerns.
-
Flexibility: The factory enables you to modify the instantiation process or add common logic in a single place without affecting the client code that uses the factory. This enhances flexibility and maintainability.
-
Simplicity: Creating instances through a factory reduces redundancy in the client code by abstracting away the instantiation details. This leads to cleaner and more concise code.
-
Centralization: All object creation logic is contained within the factory, making it easier to manage, update, and extend the creation process for various classes.
-
Dynamic Creation: By incorporating class mappings or identifiers, the factory can provide dynamic instance creation based on runtime conditions or configurations.
-
Extensibility: As the application evolves, adding new classes to the factory is straightforward. The factory can be updated to accommodate new classes without modifying existing code.
-
Error Handling: The factory can handle cases where a class does not exist or when instantiation fails, providing better error handling and reducing the likelihood of runtime errors.
Conclusion:
This approach allows you to extend the factory with new classes without modifying the factory class itself, making your code more open to changes and easier to maintain.Whether you have a few classes or a complex hierarchy of objects, an instance factory pattern promotes modular and maintainable code. It encapsulates the object creation process and abstracts away the details, allowing developers to focus on the overall functionality of their application while ensuring consistent and reliable instance creation. By adopting this pattern, you can build more robust and adaptable software systems that are easier to extend and maintain over time.
Related Keywords:
What is the best example of factory method?
Factory Method Design Pattern - Definition & Examples
What is the factory pattern to create different objects?
How to implement factory class
Usage of Factory Design Pattern
Factory Design Pattern
Support our IDKBlogs team
Creating quality content takes time and resources, and we are committed to providing value to our
readers.
If you find my articles helpful or informative, please consider supporting us financially.
Any amount (10, 20, 50, 100, ....), no matter how small, will help us continue to produce
high-quality content.
Thank you for your support!
Thank you
I appreciate you taking the time to read this article. The more that you read, the more things you will know. The more that you learn, the more places you'll go.
If you’re interested in Node.js or JavaScript this link will help you a lot.
If you found this article is helpful, then please share this article's link to your friends to whom this is required, you can share this to your technical social media groups also.
You can follow us on our social media page for more updates and latest article updates.
To read more about the technologies, Please
subscribe us, You'll get the monthly newsletter having all the published
article of the last month.