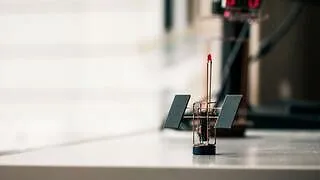
Common Coding Mistakes and How to Avoid Them with Code Example
In this article, we will explore the most common coding mistakes that developers make and provide tips on how to avoid them.
Coding is an essential skill in the digital age, powering everything from websites to mobile applications to complex enterprise systems. However, even the most experienced developers can fall victim to common coding mistakes that can cause significant issues, including bugs, crashes, and security vulnerabilities. In this article, we will explore the most common coding mistakes that developers make and provide tips on how to avoid them with code examples.1. Not Writing Secure Code
One of the most significant coding mistakes that developers make is failing to write secure code. This can result in security vulnerabilities that can be exploited by hackers, leading to data breaches and other types of cyber attacks. Common security vulnerabilities include SQL injection attacks, cross-site scripting (XSS) attacks, and buffer overflow attacks.To avoid these types of security vulnerabilities, developers should follow security best practices, such as input validation, output encoding, and parameterized queries. They should also keep their software up to date with the latest security patches and updates.
Here's an example of not writing secure code in JavaScript:
This code is meant to delete user data from a hypothetical API. However, it's not written securely because it doesn't check whether the current user is authorized to delete the data. Any user who knows another user's ID could potentially delete their data by calling this function with that ID. This is an example of an insecure direct object reference vulnerability.
To make this code more secure, we would need to implement some form of authentication and authorization. For example, we could check whether the currently logged-in user has permission to delete the requested data before making the API call. We could also implement rate limiting and other security measures to prevent abuse of the API.
2. Failing to Test Code
Another common coding mistake is failing to thoroughly test code before deploying it. This can result in bugs and other issues that can impact the functionality of the software. To avoid this mistake, developers should create comprehensive test plans and ensure that their code passes all necessary tests before deployment.Here's an example of failing to test code in JavaScript:
This code is meant to calculate the average of an array of numbers. However, it doesn't handle the case where the input array is empty, which would cause the code to throw a "divide by zero" error. This is an example of failing to test the code thoroughly and anticipate potential edge cases.
To avoid this mistake, we should always test our code with a variety of inputs, including empty inputs, to make sure it behaves correctly and doesn't produce unexpected errors. Here's an updated version of the code that includes a test for empty input:
This updated code checks whether the input array is empty and returns a value of 0 if it is, avoiding the "divide by zero" error. We should always test our code thoroughly with a variety of inputs to catch potential bugs and edge cases before deploying it to production.
3. Not Following Best Practices
Developers may also make the mistake of not following best practices when it comes to coding. This can include failing to use appropriate design patterns, not commenting their code, or not using version control systems.To avoid this mistake, developers should adhere to best practices for coding, including using appropriate design patterns, commenting code, and using version control systems like Git.
here's an example of not following best practices in JavaScript:
This code is using a single equals sign (=) instead of a double equals sign (==) or a triple equals sign (===) in the conditional statement. This is an example of not following best practices for comparison operators in JavaScript. When we use a single equals sign in a conditional statement, it assigns the value on the right-hand side to the variable on the left-hand side, so the condition always evaluates to true.
To follow best practices for comparison operators in JavaScript, we should always use double equals (==) or triple equals (===) for equality comparisons. Here's an updated version of the code that uses triple equals:
This updated code uses triple equals (===) to check if x is equal to 10. Triple equals is a strict equality comparison operator that checks both the value and the type of the operands, making it more reliable and less error-prone than double equals (==) or single equals (=). By following best practices for comparison operators, we can avoid bugs and ensure our code is more robust and maintainable.
4. Using Global Variables
One of the most common coding mistakes made by developers is using global variables. Global variables can cause issues in larger codebases, as they can be accessed and modified by any part of the program, making it difficult to track changes and potential bugs.To avoid this mistake, developers should use local variables instead of global variables. Local variables are only accessible within the scope of the function they are defined in, making it easier to track changes and debug issues.
Code Example:
Here's an example of how to use local variables instead of global variables in JavaScript:
In this example, we define a function that takes two parameters and calculates their sum. We declare a local variable "sum" inside the function, which is only accessible within the function's scope.
5. Not Using Proper Error Handling Techniques
Another common mistake made by developers is not handling errors properly. Errors can occur in software development, and failing to handle them can result in program crashes or incorrect behavior.To avoid this mistake, developers should use try-catch blocks to catch and handle errors in their code.
Code Example:
Here's an example of how to use a try-catch block to handle errors in JavaScript:
In this example, we use a try-catch block to catch and handle any errors that may occur in the code. If an error occurs, it will be caught and logged to the console.
6. Not Optimizing Code
Developers may also make the mistake of not optimizing their code, resulting in slow performance and inefficient code. This can be a significant issue in larger projects or applications.To avoid this mistake, developers should optimize their code by using best practices, such as minimizing function calls and using efficient algorithms.
Code Example:
Here's an example of how to optimize code in JavaScript by minimizing function calls:
In this example, we define a function that takes two parameters and calculates their sum. Instead of calling the function twice with different parameters, we declare the parameters as variables and call the function once, resulting in optimized code.
7. Not Declaring Variables
One common mistake that many developers make is not declaring variables. This can lead to issues such as unexpected behavior, errors, and performance problems. When a variable is not declared, JavaScript automatically creates a global variable, which can lead to naming collisions with other variables and functions.To avoid this mistake, developers should always declare variables using the var, let, or const keyword. var is used to declare variables that are scoped to the function or global object, let is used to declare variables that are scoped to the block in which they are defined, and const is used to declare variables that cannot be reassigned.
Code Example:
Here's an example of how to declare variables in JavaScript using the let keyword:
8. Not Using Strict Mode
Another common mistake is not using strict mode in JavaScript. Strict mode is a feature in JavaScript that helps to prevent common coding mistakes by throwing errors and limiting certain behaviors. When strict mode is enabled, JavaScript code must follow stricter rules, which can help to catch errors early on.To enable strict mode, developers can add the string "use strict" at the beginning of a JavaScript file or function.
Code Example:
Here's an example of how to enable strict mode in JavaScript:
In this example, the "use strict" string is added at the beginning of the file to enable strict mode.
9. Using the Wrong Equality Operator
Another common mistake that many JavaScript developers make is using the wrong equality operator. JavaScript has two equality operators, == and ===. The == operator compares two values for equality, but it does not take into account the data type of the values. The === operator, on the other hand, compares two values for equality and also checks if they are of the same data type.To avoid this mistake, developers should always use the === operator when comparing values for equality.
Code Example:
Here's an example of how to use the === operator in JavaScript:
In this example, the === operator is used to compare the values of x and y. Since x is a number and y is a string, they are not equal, and the code will output "Not equal".
10. Not Declaring Variables Properly
One of the most common coding mistakes in JavaScript is not declaring variables properly. This can lead to unintended consequences, such as overwriting existing variables or causing errors in the code.To avoid this mistake, developers should declare variables using the proper syntax, which includes the 'var', 'let', or 'const' keyword, followed by the variable name.
Code Example:
Here's an example of how to properly declare variables in JavaScript:
11. Not Checking for Undefined Values
Another common coding mistake in JavaScript is not checking for undefined values. This can lead to unexpected behavior in the code and make it difficult to debug.To avoid this mistake, developers should always check for undefined values before using them in their code.
Code Example:
Here's an example of how to check for undefined values in JavaScript:
Conclusion:
Coding mistakes can have significant consequences in software development, including bugs, crashes, and security vulnerabilities.By following best practices and using code examples, developers can avoid common coding mistakes and ensure that their code is efficient and bug-free. By taking the time to optimize code, handle errors properly, and avoid using global variables, developers can improve the performance and security of their code.
So, always strive to write clean and efficient code to avoid these mistakes.
Don't forget to subscribe to our newsletter for more useful articles on software development and coding tips.
Related Keywords:
Common coding mistakes
Avoiding coding mistakes
JavaScript coding mistakes
JavaScript best practices
Declaring variables in JavaScript
Comparing values in JavaScript
Checking for undefined values in JavaScript
Error handling in JavaScript
Support our IDKBlogs team
Creating quality content takes time and resources, and we are committed to providing value to our
readers.
If you find my articles helpful or informative, please consider supporting us financially.
Any amount (10, 20, 50, 100, ....), no matter how small, will help us continue to produce
high-quality content.
Thank you for your support!
Thank you
I appreciate you taking the time to read this article. The more that you read, the more things you will know. The more that you learn, the more places you'll go.
If you’re interested in Node.js or JavaScript this link will help you a lot.
If you found this article is helpful, then please share this article's link to your friends to whom this is required, you can share this to your technical social media groups also.
You can follow us on our social media page for more updates and latest article updates.
To read more about the technologies, Please
subscribe us, You'll get the monthly newsletter having all the published
article of the last month.