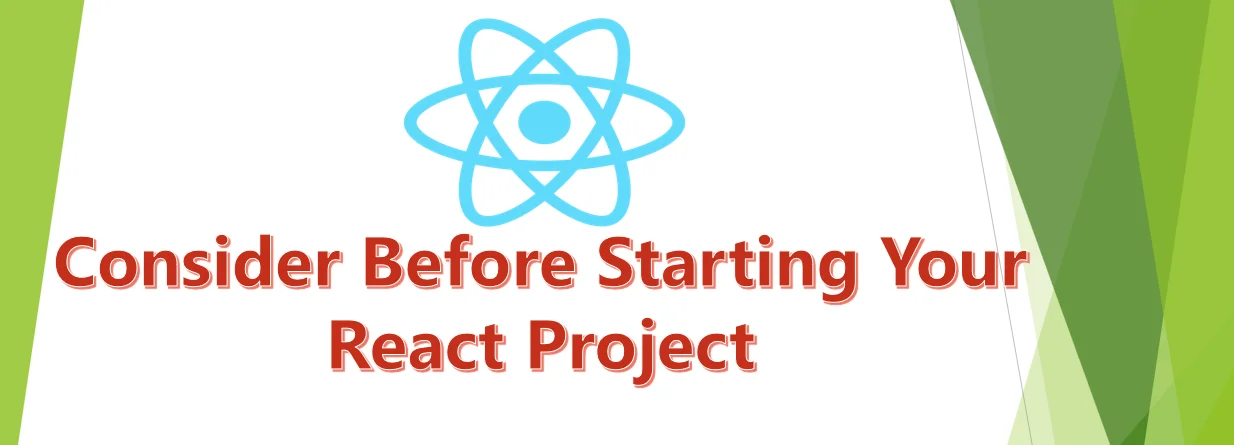
I
nternalization means adaptation of our application, product or document content to meet the different language.
Basically, Internalization is the design and development of application/product as per the easy localization for
target audiences that different in culture, language, or region.
Internalization is the technique of describing something according to the end-users in many countries
or you can say in many languages.
Here, Internalization means constructing a web app with the support of multiple languages according to the
geolocation.
In this article, we will create a react demo app and then we will implement i18n ( react-intl ) in our app and
give the multiple language support ( English, German and French ).
Let's get started and implement internalization (i18n) in react app.
Step 1: Create a React app:
To start this, we need to create a demo app using below command, let’s run the below command and create a demo app “i18n-demo”:
npx create-react-app i18n-demo
After successful completion of the above command :
cd i18n-demo
Also, let’s install the following npm packages which we will use in our app:
npm install react-intl react-select
After the above command, run "npm start":
npm start
Step 2: Open localhost:3000 in the browser:
Check your current port ( here default port is 3000 ) and confirm its working as:
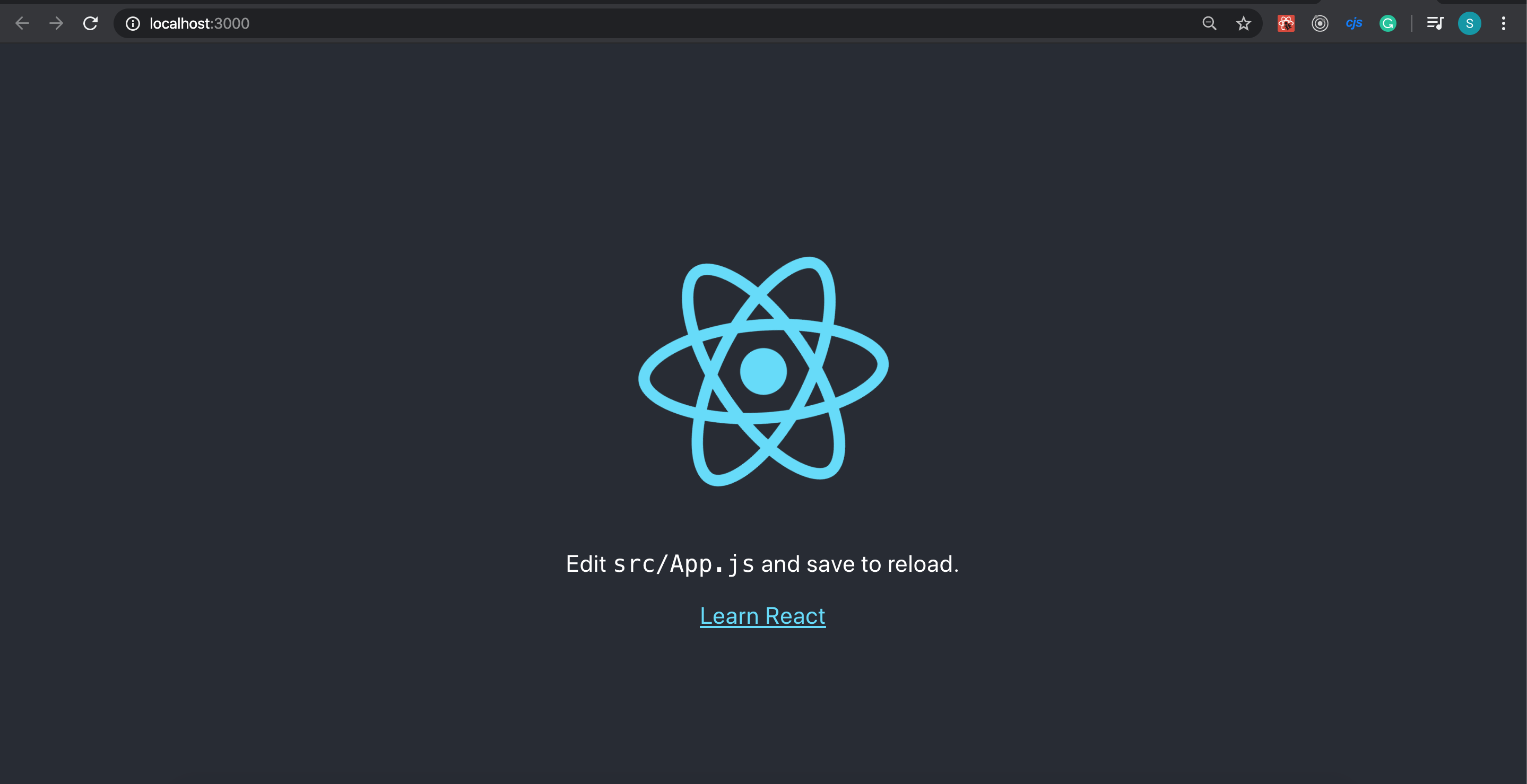
Source: idkblogs.com
Step 3: Start writing code for i18n support:
Create a folder “i18n” in the “src” folder and create a file “locales.js”. In the locales.js we keep our data like what language support we will add for our app. So let’s write the code in locales.js:
locales.js:
// locales.js
export const LOCALES = {
ENGLISH: 'en-US',
GERMAN: 'de-de',
FRENCH: 'fr-ca'
}
According to the above codes, you can see, we are going to support 3 languages in this demo. The languages are:
English
German
French
Step 4: Create a folder “messages” inside i18n folder ( src/i18n/messages) :
In this, we need to create multiple files as following:
a): Create a file “en-US.js” inside location “src/i18n/messages” and write the below code in this file:
//en-US.js
import { LOCALES } from '../locales';
export default {
[LOCALES.ENGLISH]: {
'hello': 'Hello World',
'edit': 'This example is created by {name}',
'message':'Internalization means adapting computer software to different languages. You have ability to switch between multiple languages.'
}
}
In the above codes, we are importing the supported languages from file locales.js, and we are creating our messages to display in our component and we are exporting it by default. We need to create 2 files for the other two languages ( German and French ).
b): Create a file “de-DE.js” inside location “src/i18n/messages” and write the below code :
//de-DE.js
import { LOCALES } from '../locales';
export default {
[LOCALES.GERMAN]: {
'hello': 'Hallo Welt',
'edit':'Dieses Beispiel wurde von {name} erstellt',
'message':'Internalisierung bedeutet, Computersoftware an verschiedene Sprachen anzupassen. Sie können zwischen mehreren Sprachen wechseln.'
}
}
In the above code, we imported the "LOCALES" from "locales.js". and we describe the words in german language:
eg.
"Hellow World" in german-
"Hallo Welt".
"This example is created by {name}" in german-
"Dieses Beispiel wurde von {name} erstellt".
"Internalization means adapting computer software to different languages. You have ability to switch between multiple languages." in german-
"Internalisierung bedeutet, Computersoftware an verschiedene Sprachen anzupassen. Sie können zwischen mehreren Sprachen wechseln.".
c): Create a file “fr-CA.js” inside location “src/i18n/messages” and write the below codes:
//fr-CA.js
import { LOCALES } from '../locales';
export default {
[LOCALES.FRENCH]: {
'hello': 'Bonjour le monde',
'edit':'Cet exemple est créé par {name}',
'message':'L\'internalisation signifie l\'adaptation de logiciels informatiques à différentes langues. Vous avez la possibilité de basculer entre plusieurs langues.'
}
}
In the above code, we imported the "LOCALES" from "locales.js". and we describe the words in French language:
eg.
"Hellow World" in French-
"Bonjour le monde".
"This example is created by {name}" in French-
"Cet exemple est créé par {name}".
"Internalization means adapting computer software to different languages. You have ability to switch between multiple languages." in French-
"L'internalisation signifie l\'adaptation de logiciels informatiques à différentes langues. Vous avez la possibilité de basculer entre plusieurs langues.".
Now, let’s create a file to export all the above 3 files together in “index.js”:
d): Create a file “index.js” inside message to export combined and write the below codes:
// index.js
import en from './en-US';
import de from './de-DE';
import fr from './fr-CA';
export default {
...en,
...de,
...fr
}
Step 5: Go to the “src/App.js” and delete all codes and write the below code:
Let’s understand the code first:
We are importing the necessary things for our component:
import React, { useState } from 'react';
import './App.css';
import Select from 'react-select';
import { LOCALES } from './i18n/locales';
import { FormattedMessage } from 'react-intl';
import { IntlProvider } from 'react-intl';
import messages from './i18n/messages';
In the above code:
Creating a function component App:
function App() { }
Using “useState” hook to create a state for our functional component, we are creating “locale” with the default value “en-US” and get the reference of a function to update the function.
const [locale, setLocale] = useState(LOCALES.ENGLISH);
A handler function “changeLocale” will be called when you change the selection by select dropdown:
function changeLocale(lang) {
setLocale(lang.value)
}
These are the options for dropdown:
const options = [
{ value: LOCALES.ENGLISH, label: 'English' },
{ value: LOCALES.GERMAN, label: 'Germon' },
{ value: LOCALES.FRENCH, label: 'French' },
];
The things we need to focus on are “IntlProvider” and “FormattedMessage” tags. IntlProvider is a component and it is a wrapper to wrap your root component. You need to wrap your root component to get the advantage of internalization. We need to configure it with the locales and with the translated strings or messages.
FormattedMessage is used to display the message according to the specific Id. By default, FormattedMessage renders the string into a "React.Fragment", below is the syntax of FormattedMessage:
Step 6: Run the app:
Now run the app using command
npm start
and open the browser and see the result:
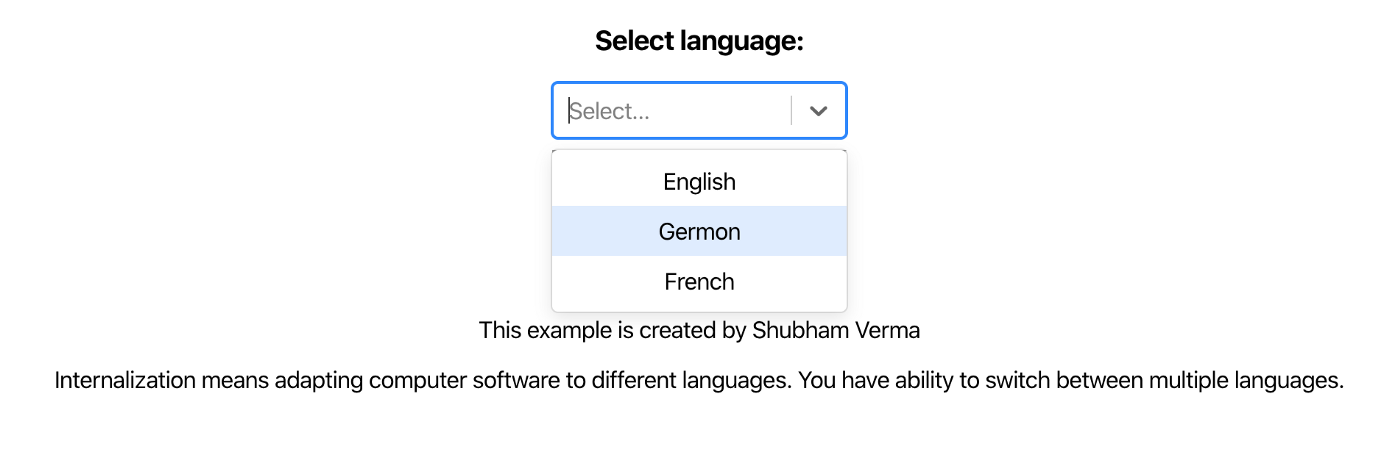
Source: idkblogs.com
You can see in the browser, our app is working file. In the browser, you can see the default language is English and when you click on the select box, you will have 3 options for choose language. after selecting the language the content of the app will be changed as in the below gif:
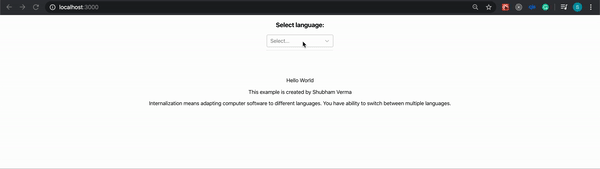
Source: idkblogs.com
Congratulation..
Conclusion:
In this article, we learned about the internalization and how we can implement internalization ( i18n ) in the
react app. Also learned how we can add the multi language support.
Now you'll be able to develop and work on i18n or internalization module in any react based application.
Support our IDKBlogs team
Creating quality content takes time and resources, and we are committed to providing value to our
readers.
If you find my articles helpful or informative, please consider supporting us financially.
Any amount (10, 20, 50, 100, ....), no matter how small, will help us continue to produce
high-quality content.
Thank you for your support!
Thank you
I appreciate you taking the time to read this article. The more that you read, the more things you will know. The more that you learn, the more places you'll go.
If you’re interested in Node.js or JavaScript this link will help you a lot.
If you found this article is helpful, then please share this article's link to your friends to whom this is required, you can share this to your technical social media groups also.
You can follow us on our social media page for more updates and latest article updates.
To read more about the technologies, Please
subscribe us, You'll get the monthly newsletter having all the published
article of the last month.